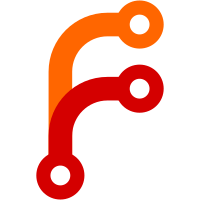
This hopefully updates all module headers such that the file encoding is utf-8, and uses Unicode literals, etc.
150 lines
4.9 KiB
Python
150 lines
4.9 KiB
Python
# -*- coding: utf-8 -*-
|
|
|
|
from __future__ import unicode_literals
|
|
|
|
from unittest import TestCase
|
|
|
|
from rattail import barcodes
|
|
|
|
|
|
class ModuleTests(TestCase):
|
|
|
|
def test_upc_check_digit(self):
|
|
data = [
|
|
('07430500132', 1),
|
|
('03600029145', 2),
|
|
('03600024145', 7),
|
|
('01010101010', 5),
|
|
('07430503112', 0),
|
|
]
|
|
for in_, out in data:
|
|
self.assertEqual(barcodes.upc_check_digit(in_), out)
|
|
|
|
def test_luhn_check_digit(self):
|
|
data = [
|
|
('7992739871', 3),
|
|
('4992739871', 6),
|
|
('123456781234567', 0),
|
|
]
|
|
for in_, out in data:
|
|
self.assertEqual(barcodes.luhn_check_digit(in_), out)
|
|
|
|
def test_price_check_digit(self):
|
|
data = [
|
|
('0512', 3),
|
|
]
|
|
for in_, out in data:
|
|
self.assertEqual(barcodes.price_check_digit(in_), out)
|
|
|
|
# short string
|
|
self.assertRaises(ValueError, barcodes.price_check_digit, '123')
|
|
|
|
# long string
|
|
self.assertRaises(ValueError, barcodes.price_check_digit, '12345')
|
|
|
|
# not a string
|
|
self.assertRaises(ValueError, barcodes.price_check_digit, 1234)
|
|
|
|
def test_upce_to_upca(self):
|
|
|
|
# without input check digit
|
|
data = [
|
|
('123450', '01200000345'),
|
|
('123451', '01210000345'),
|
|
('123452', '01220000345'),
|
|
('123453', '01230000045'),
|
|
('123454', '01234000005'),
|
|
('123455', '01234500005'),
|
|
('123456', '01234500006'),
|
|
('123457', '01234500007'),
|
|
('123458', '01234500008'),
|
|
('123459', '01234500009'),
|
|
('654321', '06510000432'),
|
|
('425261', '04210000526'),
|
|
('234903', '02340000090'),
|
|
('234567', '02345600007'),
|
|
('234514', '02345000001'),
|
|
('639712', '06320000971'),
|
|
('867933', '08670000093'),
|
|
('913579', '09135700009'),
|
|
('421184', '04211000008'),
|
|
]
|
|
for in_, out in data:
|
|
self.assertEqual(barcodes.upce_to_upca(in_), out)
|
|
|
|
# with input check digit
|
|
data = [
|
|
('01234505', '01200000345'),
|
|
('01234514', '01210000345'),
|
|
('01234523', '01220000345'),
|
|
('01234531', '01230000045'),
|
|
('01234543', '01234000005'),
|
|
('01234558', '01234500005'),
|
|
('01234565', '01234500006'),
|
|
('01234572', '01234500007'),
|
|
('01234589', '01234500008'),
|
|
('01234596', '01234500009'),
|
|
('06543217', '06510000432'),
|
|
('04252614', '04210000526'),
|
|
('02349036', '02340000090'),
|
|
('02345673', '02345600007'),
|
|
('02345147', '02345000001'),
|
|
('06397126', '06320000971'),
|
|
('08679339', '08670000093'),
|
|
('09135796', '09135700009'),
|
|
('04211842', '04211000008'),
|
|
]
|
|
for in_, out in data:
|
|
self.assertEqual(barcodes.upce_to_upca(in_), out)
|
|
|
|
# with output check digit
|
|
data = [
|
|
('123450', '012000003455'),
|
|
('123451', '012100003454'),
|
|
('123452', '012200003453'),
|
|
('123453', '012300000451'),
|
|
('123454', '012340000053'),
|
|
('123455', '012345000058'),
|
|
('123456', '012345000065'),
|
|
('123457', '012345000072'),
|
|
('123458', '012345000089'),
|
|
('123459', '012345000096'),
|
|
('654321', '065100004327'),
|
|
('425261', '042100005264'),
|
|
('234903', '023400000906'),
|
|
('234567', '023456000073'),
|
|
('234514', '023450000017'),
|
|
('639712', '063200009716'),
|
|
('867933', '086700000939'),
|
|
('913579', '091357000096'),
|
|
('421184', '042110000082'),
|
|
]
|
|
for in_, out in data:
|
|
self.assertEqual(
|
|
barcodes.upce_to_upca(in_, include_check_digit=True), out)
|
|
|
|
# with input and output check digits
|
|
data = [
|
|
('01234505', '012000003455'),
|
|
('01234514', '012100003454'),
|
|
('01234523', '012200003453'),
|
|
('01234531', '012300000451'),
|
|
('01234543', '012340000053'),
|
|
('01234558', '012345000058'),
|
|
('01234565', '012345000065'),
|
|
('01234572', '012345000072'),
|
|
('01234589', '012345000089'),
|
|
('01234596', '012345000096'),
|
|
('06543217', '065100004327'),
|
|
('04252614', '042100005264'),
|
|
('02349036', '023400000906'),
|
|
('02345673', '023456000073'),
|
|
('02345147', '023450000017'),
|
|
('06397126', '063200009716'),
|
|
('08679339', '086700000939'),
|
|
('09135796', '091357000096'),
|
|
('04211842', '042110000082'),
|
|
]
|
|
for in_, out in data:
|
|
self.assertEqual(
|
|
barcodes.upce_to_upca(in_, include_check_digit=True), out)
|